Basically, I wanted to draw a waveform like Sound Forge or Wavelab does, 1 pixel thick:
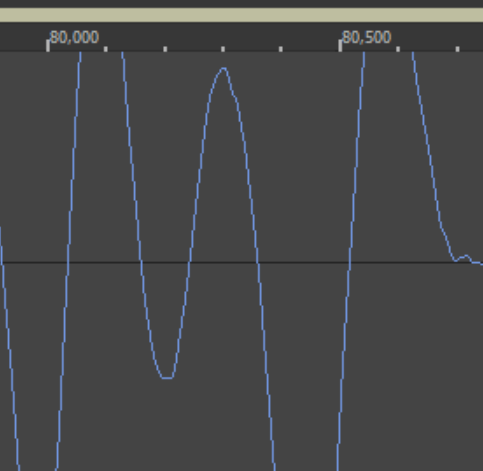
You can't get this with Bresenham, instead, you only draw vertical lines with this logic:
if (max < next.Min)
{
max = next.Min;
}
if (min > next.Max)
{
min = next.Max;
}
So far, this work on a good old 2D surface, but with OpenGL it ended up being an impossible mission…
Wrote a small program to try understand this insanity and inferred the following:
// Lines EXACTLY 1 pixel thick, with or without MSAA:
//
// 1x1 square:
//
// X1 = 10.0
// Y1 = 10.0
// X2 = 11.0
// Y2 = 11.0
//
// 2x1 horizontal:
//
// X1 = 20.0
// Y1 = 20.5
// X2 = 22.0
// Y2 = 20.5
//
// 1x2 vertical:
//
// X1 = 30.5
// Y1 = 30.0
// X2 = 30.5
// Y2 = 32.0
//
// 2x2 diagonal:
//
// X1 = 40.0
// Y1 = 40.0
// X2 = 42.0
// Y2 = 42.0
This works but that's theory, reality is different. Turns out it's impossible to get 1 pixel thick lines working all the time.
Wrote another test program:
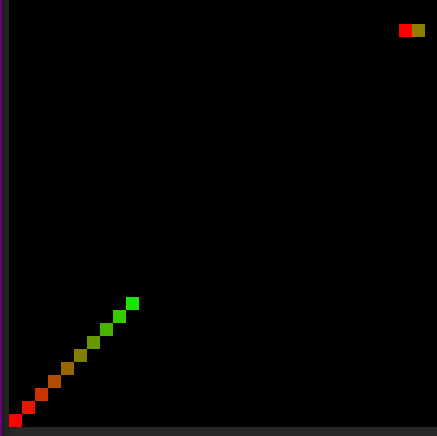
Notice how the 2nd line end pixel isn't green…
If you make the line 1.1 pixel wide!, you get green although it's not the end color…
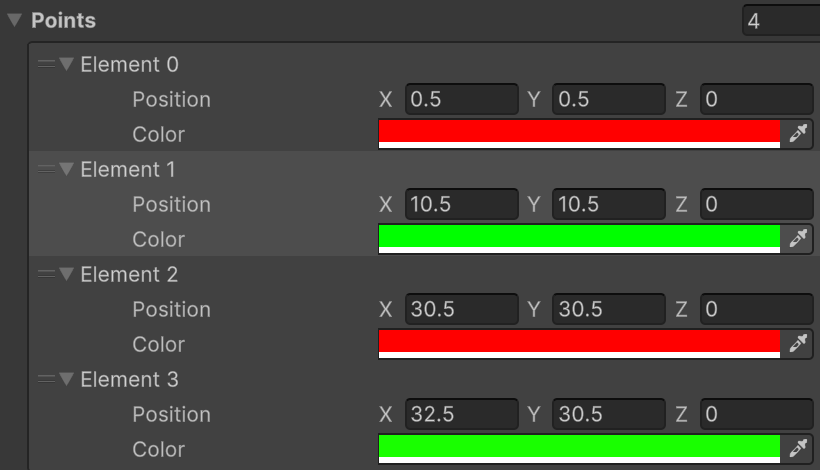
Also, you simply cannot use GL_LINES, you must use GL_LINESTRIP else you have empty lines:
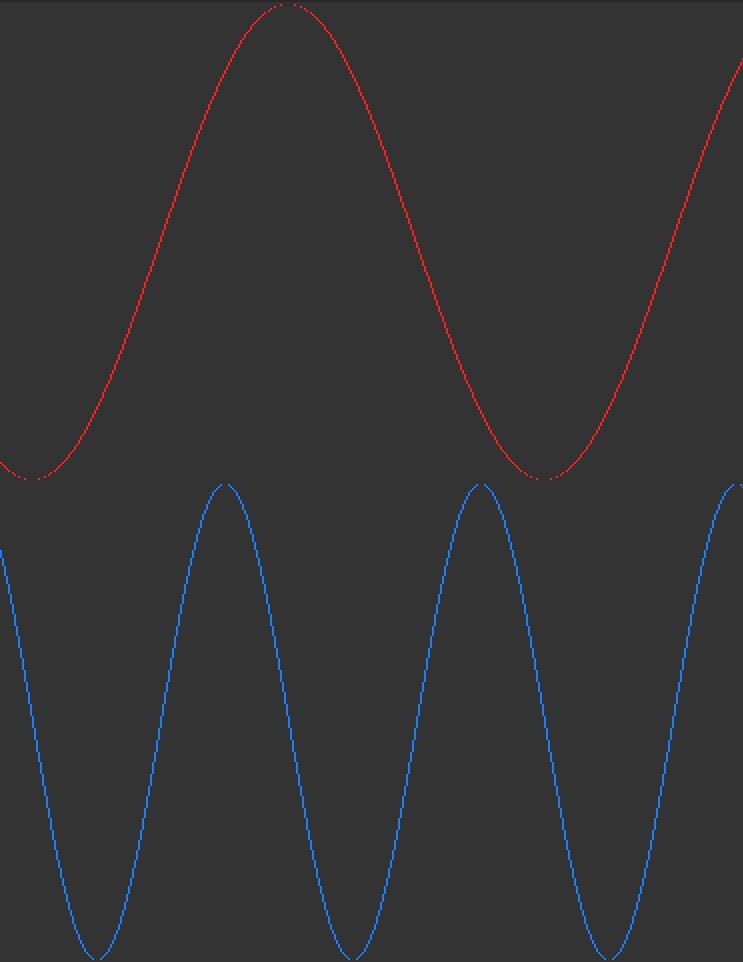
But then GL_LINESTRIP thickness isn't even, unless one goes 4K it's noticeable:
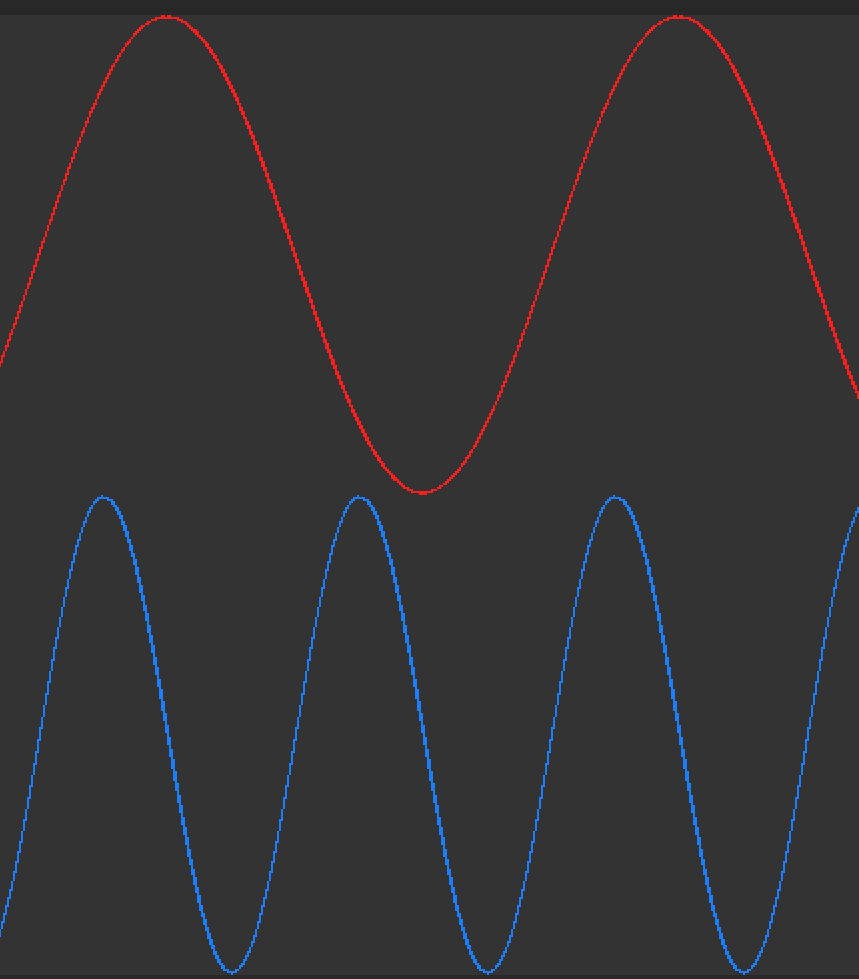
Trying to enable MSAA simply moves the problem somewhere else + free artifacts.
Tried tons of things:
- adding +/-0.5 offsets, turns out for Y axis it's a no go
- ensuring a Y1 ≠ Y2 so that lines are always visible, not good overall
- wrote a shader that ‘undo’ MSAA pixels, it works but to some extent only
- wrote a 2D surface that does Bresenham with Burst, works, is fast but chokes on 4K…
- looked at rasterization rules GL and DX (hey I just wanted to draw lines)
What have we become? We have RTX 4050 my ass but can't instruct it to draw precise 2D lines!!??
Can do that in QBASIC in like 30 seconds, it just works...
I am not crazy, right? Or am I?
Any clues are welcome, thanks!