Hi,
I'm getting back into C++ programming (and game development). I learnt C++ pre C++11, so I'm trying to pick up the new stuff.
I've been looking into memory management of game objects, with a focus of laying game objects out in memory efficiently. I've come across the std::pmr::monotonic_buffer_resource
, where you supply it a region of memory for it to use:
union Vec2 {
struct {
float x;
float y;
};
struct {
float w;
float h;
};
float elements[2];
};
struct GameObject {
Vec2 position;
Vec2 size;
GameObject() : position{ 0,0 }, size{ 0,0 } {};
GameObject(Vec2 _position, Vec2 _size) : position(_position), size(_size) {};
};
int main() {
GameObject buffer[256];
const auto GameObjectPrinter = [](const GameObject& gameObject, std::string_view title) {
std::cout << title << '\n';
std::cout << "Position: x = " << gameObject.position.x << ", y = " << gameObject.position.y << '\n';
std::cout << "Size: w = " << gameObject.size.w << ", h = " << gameObject.size.h << '\n';
std::cout << '\n';
};
std::pmr::monotonic_buffer_resource pool{ std::data(buffer), std::size(buffer) };
std::pmr::vector<GameObject> vec{ &pool };
vec.reserve(5);
vec.emplace_back(GameObject({ 0, 0 }, { 32, 32 }));
vec.push_back({ {1, 1}, {16, 16} });
for (auto& item : vec) {
GameObjectPrinter(item, "Game Objects:");
}
}
On the surface this seems to work fine. However, I was curious and wanted to see what was happening under the hood, and how it was using buffer
. Debugging it in Visual Studio shows that the vector puts it's first element in buffer[1] rather than buffer[0], does anyone know why this is (it's more than likely something I have done wrong and I can't see it)?
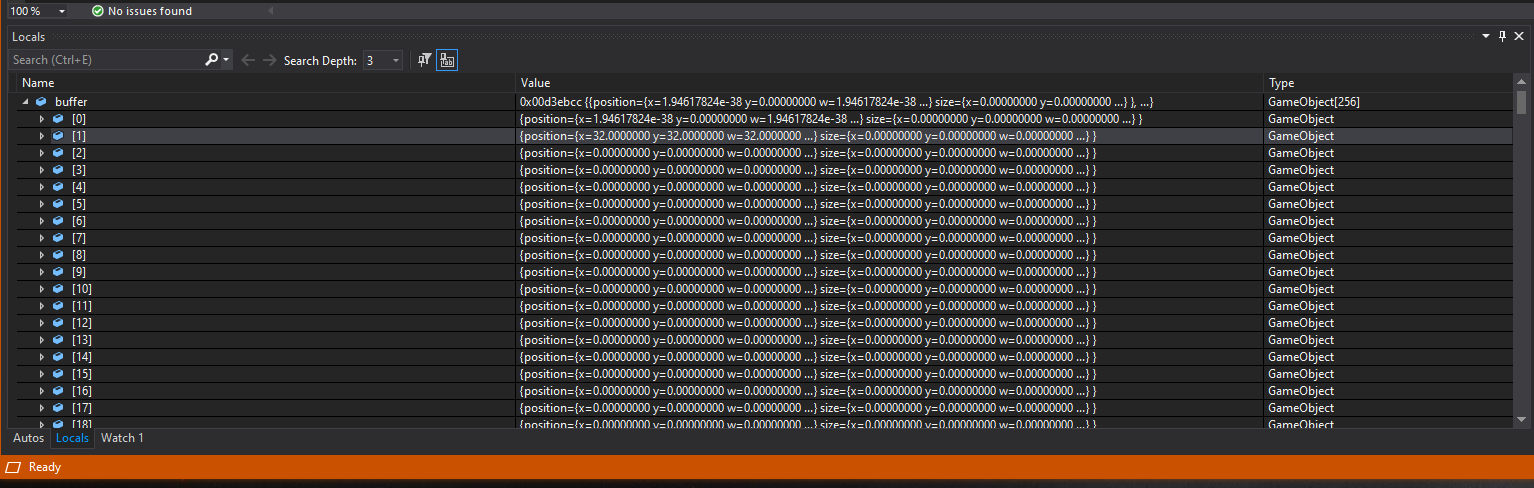