Hello, i am trying to render a model with a texture that is transparent, i got transparency to work, however when i look through the part of the model that is transparent, i can't see what is behind it, it just cuts off everything except skybox that is behind it, an example shown here:
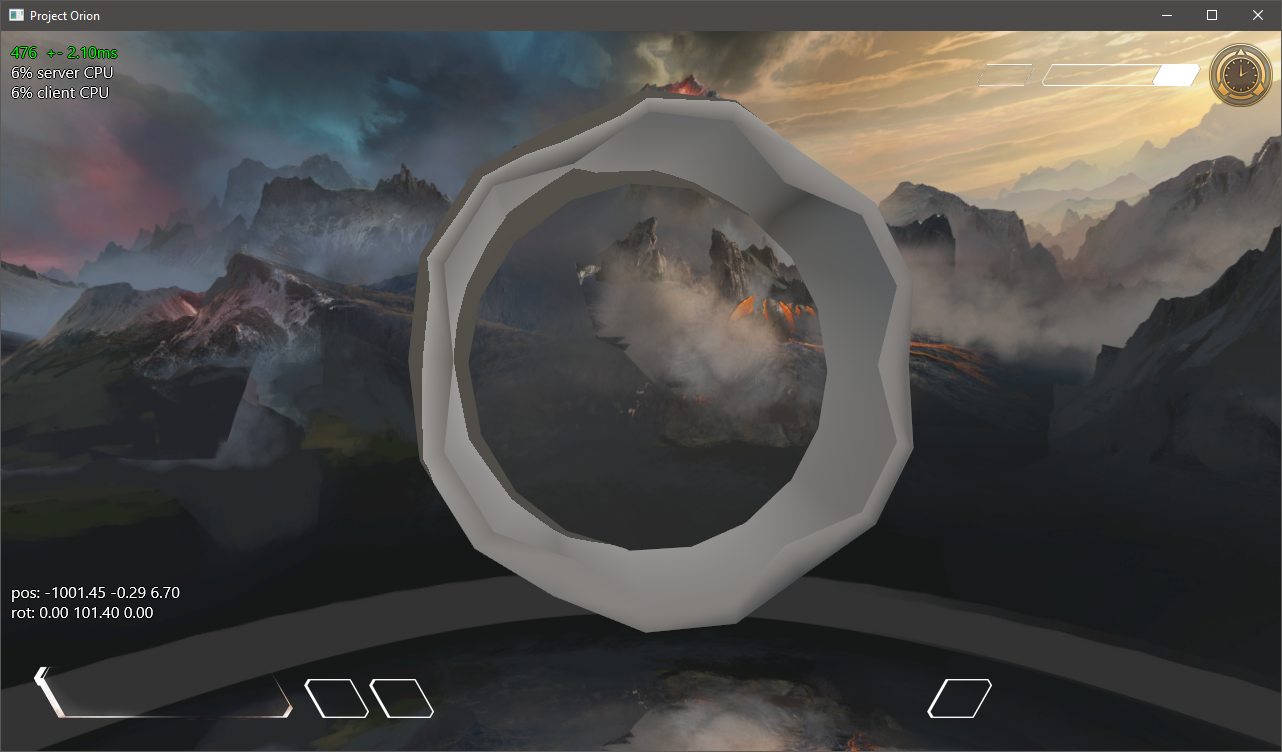
I am not sure what is causing it, perhaps blend state? Here is my blend state description:
D3D11_BLEND_DESC blendStateDesc;
ZeroMemory(&blendStateDescription, sizeof(D3D11_BLEND_DESC));
blendStateDesc.RenderTarget[0].BlendEnable = TRUE;
blendStateDesc.RenderTarget[0].SrcBlend = D3D11_BLEND_SRC_ALPHA;
blendStateDesc.RenderTarget[0].DestBlend = D3D11_BLEND_INV_SRC_ALPHA;
blendStateDesc.RenderTarget[0].BlendOp = D3D11_BLEND_OP_ADD;
blendStateDesc.RenderTarget[0].SrcBlendAlpha = D3D11_BLEND_ONE;
blendStateDesc.RenderTarget[0].DestBlendAlpha = D3D11_BLEND_ZERO;
blendStateDesc.RenderTarget[0].BlendOpAlpha = D3D11_BLEND_OP_ADD;
blendStateDesc.RenderTarget[0].RenderTargetWriteMask = 0x0f;
m_Device11->CreateBlendState(&blendStateDesc, &m_BlendStateEnable11);
float blendColor[4];
blendColor[0] = 0.0f;
blendColor[1] = 0.0f;
blendColor[2] = 0.0f;
blendColor[3] = 0.0f;
m_DeviceContext11->OMSetBlendState(m_BlendStateEnable11, blendColor, 0xffffffff);
Here is the full code of draw calls:
float bgColor[4] = { (0.0f, 0.0f, 0.0f, 0.0f) };
m_DeviceContext11->ClearRenderTargetView(m_FrameBuffer11.renderTargetView11, bgColor);
m_DeviceContext11->ClearDepthStencilView(m_FrameBuffer11.depthStencilView11, D3D11_CLEAR_DEPTH | D3D11_CLEAR_STENCIL, 1.0f, 0);
...
m_Interface->m_Tier0->m_DirectX11->m_DeviceContext11->OMSetRenderTargets(1, &m_Interface->m_Tier0->m_DirectX11->m_FrameBuffer11.renderTargetView11, m_Interface->m_Tier0->m_DirectX11->m_FrameBuffer11.depthStencilView11);
m_Interface->m_Tier0->m_DirectX11->m_DeviceContext11->IASetPrimitiveTopology(D3D11_PRIMITIVE_TOPOLOGY_TRIANGLELIST);
float blendColor[4];
blendColor[0] = 0.0f;
blendColor[1] = 0.0f;
blendColor[2] = 0.0f;
blendColor[3] = 0.0f;
m_Interface->m_Tier0->m_DirectX11->m_DeviceContext11->OMSetBlendState(m_Interface->m_Tier0->m_DirectX11->m_BlendStateEnable11, blendColor, 0xffffffff);
m_Interface->m_Tier0->m_DirectX11->m_DeviceContext11->RSSetState(m_Interface->m_Tier0->m_DirectX11->m_RasterizerStateClockwise11);
m_Interface->m_Tier0->m_DirectX11->m_DeviceContext11->VSSetConstantBuffers(0, 1, &m_Interface->m_Tier0->m_DirectX11->m_TransformBuffer11);
m_GlfwLoader->DrawModel();
m_Interface->m_Tier0->m_DirectX11->m_DeviceContext11->IASetVertexBuffers(0, 1, &m_VertexBuffer, &stride, &offset);
m_Interface->m_Tier0->m_DirectX11->m_DeviceContext11->IASetIndexBuffer(m_IndexBuffer, DXGI_FORMAT_R32_UINT, 0);
...
m_Interface->m_Tier0->m_DirectX11->m_DeviceContext11->VSSetShader(m_Interface->m_Tier0->m_DirectX11->m_Shader11->m_DefaultShader11->m_VertexShader11, nullptr, 0);
m_Interface->m_Tier0->m_DirectX11->m_DeviceContext11->PSSetShader(m_Interface->m_Tier0->m_DirectX11->m_Shader11->m_DefaultShader11->m_PixelShader11, nullptr, 0);
m_Interface->m_Tier0->m_DirectX11->m_DeviceContext11->PSSetShaderResources(0, 7, shaderResourceViews);
m_Interface->m_Tier0->m_DirectX11->m_DeviceContext11->DrawIndexed(static_cast<UINT>(m_Indices.size()), 0, 0);
And in case if there's something wrong in the shader code, there it is:
Texture2D albedoTexture : register(t0);
float4 main_ps(PixelShaderInput pin) : SV_Target
{
float3 albedo = albedoTexture.Sample(defaultSampler, pin.texcoord).rgb;
...
return float4(directLighting + ambientLighting, albedoTexture.Sample(defaultSampler, pin.texcoord).a);
}