Hello all! I've managed to render multiple scenes and split them into 4 “monitors" similar to CCTV thanks to help from you all.
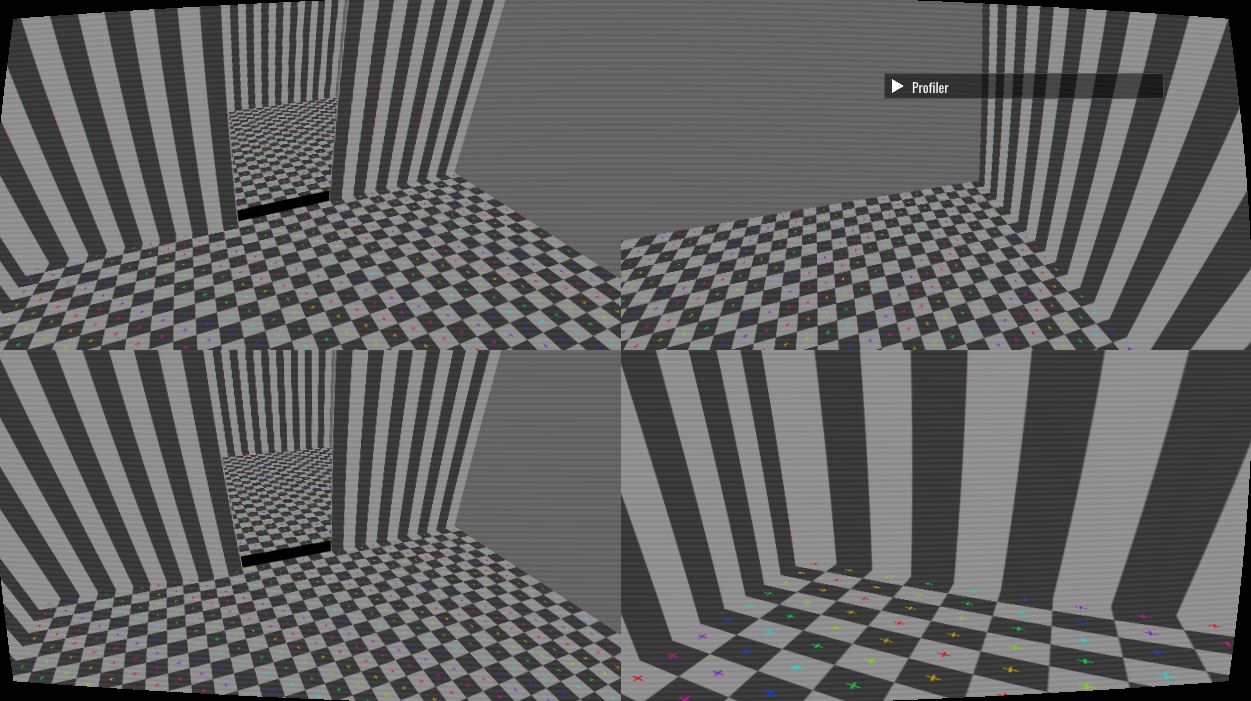
However, my post processing effects are of course rendering at full screen. Effects like vignette or just curving the screen are rendering at window size. Hopefully you can notice the window split in 4 screens though.
Is there a way I can specify the viewport x,y, width, height but in GLSL? For example, I got a curve function from shader toy:
vec2 curve(vec2 uv) {
uv = (uv - 0.5) * 2.0;
uv *= 1.1;
uv.x *= 1.0 + pow((abs(uv.y) / 5.0), 2.0);
uv.y *= 1.0 + pow((abs(uv.x) / 4.0), 2.0);
uv = (uv / 2.0) + 0.5;
uv = uv *0.92 + 0.04;
return uv;
}
and to make it work I just replace the current uv vec2 with one calculated by the curve function:
vec2 uvs = curve( uv );
What I'm trying to do is apply the curve effect to all 4 screens. I have no idea how to do this :( What if I wanted to add a glitch effec to each one? I hope my question made sense, not good with words.
Thanks all, always helpful!
Btw, I'm sure you all know this, but just in case: I'm using 1 FBO to record the scene and render the scene texture to a quad that takes up the screen, which is what yous see above.